Hey everyone! My name is Ajay Venkat I am a programmer living in Australia. I am currently developing a video game called Crash which is a RTS battle game where you can command hundreds of units through code!
This is what the arena looks like.
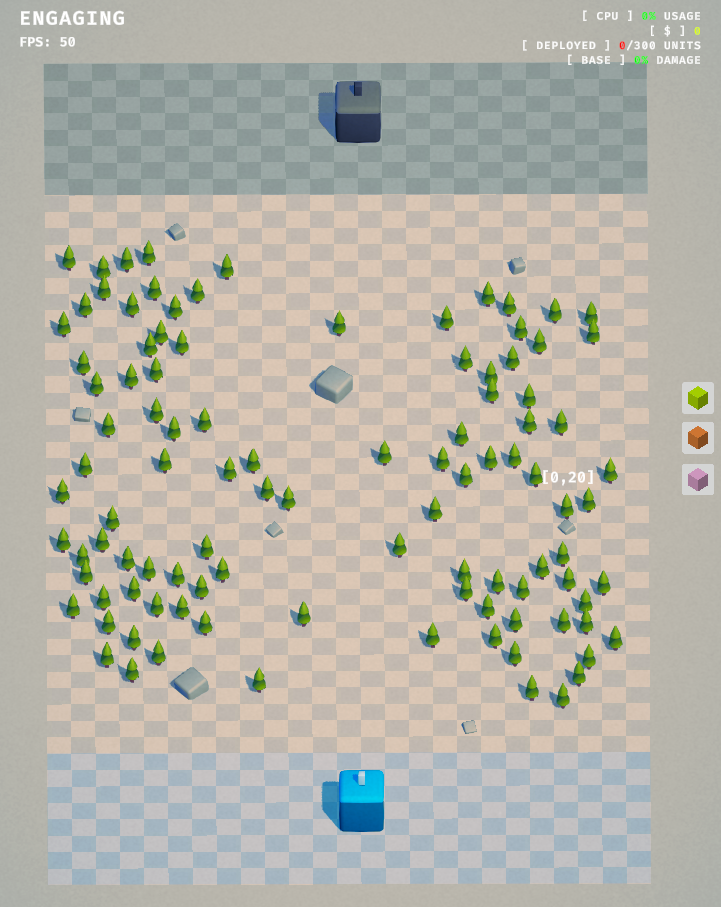
The objective is simple, you have to destroy the enemy base. To play all you have to do is open up any of your favourite code editors, open up a Python file and import a module called crash
and you’re off!
An example of the type of code you can write:
from crash import *
import math
kill = True
async def kill_in_5():
global kill
await seconds(5)
kill = False
async def move_weird(archer: Archer):
global kill
while kill:
x = math.cos(game.clock.game_time*5)
y = math.sin(game.clock.game_time*5)
archer.move(x,y)
await ticks(2)
async def begin_attack(game: Game, u: Unit):
while True:
u = u.as_soldier()
enemy_len = len(game.unit_manager.alive_enemies())
print("Enemies : " + str(enemy_len), end='\r')
if enemy_len > 0:
e = game.unit_manager.closest_enemy(u)
while not e.dead:
#print(e.unit_id)
pos = e.position
u.move_to(pos.x, pos.z)
await until(lambda : u.position.distance_to(pos) < u.attack_range or e.dead)
u.attack(pos)
await until(lambda : u.attack_timeout <= 0 or e.dead)
else:
u.stop_movement()
print("All enemies destroyed, go in circles...", end='\r')
await move_weird(game, u)
await tick()
break
def start(game : Game):
for i, u in enumerate(game.unit_manager.units_of_type(Soldier)):
game.run_background(begin_attack(game, u))
for i, u in enumerate(game.unit_manager.units_of_type(Archer)):
game.run_background(move_weird(game, u))
pass
def infinite(game : Game):
pass
def finish(game: Game):
print("Game has finished", end='\r')
game.unit_manager.stop_all_units()
pass
start_game(start, infinite, finish)
When you hit play, this will auto-connect to the game client and start executing your code against your enemies.
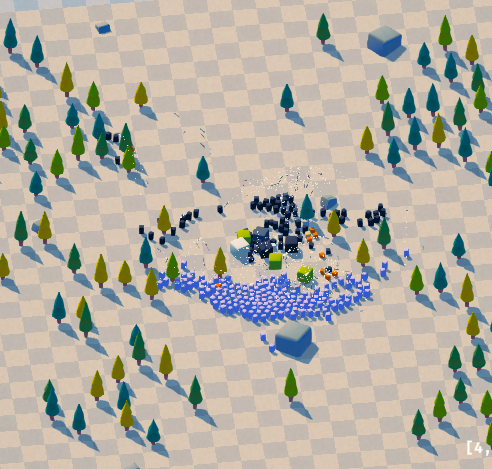
The current supported game mode is Sandbox however I aim to support Multiplayer in the coming months.
There is a lot to explain about how all of this works, and I’m really excited to finally start sharing about the game. I will also be posting development logs regularly on my YouTube Channel.
I am starting to build a community so I will be posting a Discord channel soon, everyone is welcome to come test and play the game with me once I finish the development of multiplayer!
See you next time!